Implementation of Redis Cache in C#
This post is about how to implement Redis Cache in NET Core Web API. Using Redis cache with .NET Core is a powerful way to boost the performance and scalability of your applications. Redis provides a fast and efficient in-memory data store that can be used to cache frequently accessed data, reducing the load on your primary data store and improving overall responsiveness.
Let’s start first with the installation of the Redis-x64-3.0.504.msi package using the Redis Package link.
Note: The following example is based on the .NET 8.0 version.
Step 1: Install StackExchange.Redis via NuGet Package Manager. If you want to use NuGet, just search for “StackExchange.Redis” or run the following command in the NuGet Package Manager console:
PM>NuGet\Install-Package StackExchange.Redis -Version 2.7.33
This command is intended to be used within the Package Manager Console in Visual Studio, as it uses the NuGet module's version of Install-Package.
Step 2: Once the installation is done, we need to add the Redis cache service interface.
public interface IRedisCacheService
{
T GetData(string key);
bool SetData(string key, T value, DateTimeOffset expirationTime);
object RemoveData(string key);
}
Step 3: Once the interface has been added, we now need to add the Redis cache service class.
public class RedisCacheService : IRedisCacheService
{
private IDatabase _db;
public RedisCacheService()
{
ConfigureRedis();
}
private void ConfigureRedis()
{
_db = ConnectionHelper.Connection.GetDatabase();
}
public T GetData(string key)
{
var value = _db.StringGet(key);
if (!string.IsNullOrEmpty(value))
{
return JsonConvert.DeserializeObject(value);
}
return default;
}
public object RemoveData(string key)
{
bool _isKeyExist = _db.KeyExists(key);
if (_isKeyExist == true)
{
return _db.KeyDelete(key);
}
return false;
}
public bool SetData(string key, T value, DateTimeOffset expirationTime)
{
TimeSpan expiryTime = expirationTime.DateTime.Subtract(DateTime.Now);
var isSet = _db.StringSet(key, JsonConvert.SerializeObject(value), expiryTime);
return isSet;
}
}
Step 4: We are going to add the ConnectionHelper class under the Helper folder.
public class ConnectionHelper
{
static ConnectionHelper()
{
ConnectionHelper.lazyConnection = new Lazy(() =>
{
return ConnectionMultiplexer.Connect(ConfigurationManager.AppSetting["RedisURL"]);
});
}
private static Lazy lazyConnection;
public static ConnectionMultiplexer Connection
{
get
{
return lazyConnection.Value;
}
}
}
Step 5: Add the following line of code to get data from the cache service class:
[ApiController]
[Route("[controller]")]
public class BlogController : ControllerBase
{
private static readonly string[] Summaries =
[
"Java", "C#", "C++", "SQL Server"
];
private readonly ILogger _logger;
private readonly IRedisCacheService _cacheService;
public BlogController(ILogger logger,
IRedisCacheService cacheService)
{
_logger = logger;
_cacheService = cacheService;
}
[HttpGet(Name = "GetBlog")]
public IEnumerable Get()
{
var cacheData = _cacheService.GetData>("blog");
if (cacheData != null)
{
return cacheData;
}
var expirationTime = DateTimeOffset.Now.AddMinutes(5.0);
cacheData = Enumerable.Range(1, 5).Select(index => new BlogModel
{
Stock = Random.Shared.Next(-20, 55),
BlogId = Random.Shared.Next(-20, 55),
BlogDescription = Summaries[Random.Shared.Next(Summaries.Length)],
BlogName = Summaries[Random.Shared.Next(Summaries.Length)],
BlogSummary = Summaries[Random.Shared.Next(Summaries.Length)]
}).ToList();
_cacheService.SetData>("blog", cacheData, expirationTime);
return cacheData;
}
}
Run your .NET Web API application and test the Redis cache by accessing the endpoints that use caching. Monitor the Redis server to observe caching behavior and verify performance improvements. By following these steps, you can seamlessly integrate Redis cache into your .NET Web API application, improving performance and scalability by leveraging Redis's high-speed, in-memory data store.
Download Source Code: GitHub Repository
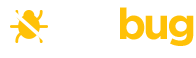