What is Scriban and how to use it in C#
A template engine allows us to use static template files, and at runtime replaces variables in a template file with the C# variables and objects, into an HTML file sent to the client. Traditionally, we used placeholders in .Net to replace text with dynamic values for example if we want to send an email, we will design a static email template with placeholders {replace text} and dynamically set its values.
Let’s start first with the installation of the Scriban library in the .NET framework.
First, Install Scriban via NuGet Package Manager. If you want to use NuGet, just search for “Scriban” or run the following command in the NuGet Package Manager console:
PM> Install-Package Scriban -Version 5.7.0
This command is intended to be used within the Package Manager Console in Visual Studio, as it uses the NuGet module's version of Install-Package.
Scriban uses the language, like a liquid template. This means it’s a combination of Objects, Tags, and filters inside template files to display dynamic content.
Object means it’s containing the content enclosed in double curly braces: {{and}}
var subject = "CRM";
var tpl = Template.Parse("Ticket is Assigned to {{subject}}!");
var res = tpl.Render(new { subject = subject });
Console.WriteLine(res);
// output
// Ticket is Assigned to CRM
Tags allow us to write the logic and conditions or loops using the curly brace percentage delimiters {{- and -}}.
1) For Loop - Implement for loop in the template using Scriban:
string[] countries = { "usa", "uk", "canada", "denmark", "australia" };
var static_html = @"
<ul>
{{- for country in countries }}
<li> {{ country }} </li>
{{- end }}
</ul>
";
2) IF/Else - Implement for loop in the template using Scriban:
string?[] countries = { "usa", "uk", "canada"};
var data = @"
{{- for country in countries -}}
{{ if country == 'usa' }}
Found {{country}}!
{{ else }}
Not Found !
{{ end }}
{{- end }}";
var tpl = Template.Parse(data);
var res = tpl.Render(new { countries = countries });
Console.WriteLine(res);
//output
// Found usa!
// Not Found!
// Not Found!
If you want to study more about the Liquid template, kindly Click Here !!
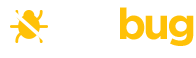