Web API token based authentication example c# step by step
This post is about how to implement JWT(JSON web token) authentication in.NET 8.0. Let's first understand what authentication is. Authentication provides a framework for secure delegation of authorization. It enables users to grant access to their resources from one website to another without sharing their credentials. OAuth operates through a series of interactions between three parties: the resource owner (user), the client (application), and the authorization server.
Adding JWT auth to the.NET 8.0 Application.
Note: The following example is based on the .NET 8.0 version.
Step 1: Install Microsoft.IdentityModel.Tokens via NuGet Package Manager. If you want to use NuGet, just search for “Microsoft.IdentityModel.Tokens” or run the following command in the NuGet Package Manager console:
PM> Install-Package Microsoft.IdentityModel.Tokens -Version 7.5.1
PM> Install-Package System.IdentityModel.Tokens.Jwt -Version 7.5.1
PM> Install-Package Microsoft.AspNetCore.Authentication.JwtBearer -Version 8.0.4
This command is intended to be used within the Package Manager Console in Visual Studio, as it uses the NuGet module's version of Install-Package.
Step 2: Once the installation is done, we need to add the following line of code in the startup.cs file.
builder.Services.AddSwaggerGen(option =>
{
option.SwaggerDoc("v1", new OpenApiInfo { Title = "JWTToken", Version = "v1", Description = "An ASP.NET Core Web API for JWTToken" });
option.AddSecurityDefinition("Bearer", new OpenApiSecurityScheme
{
In = ParameterLocation.Header,
Description = "Please enter a valid token",
Name = "Authorization",
Type = SecuritySchemeType.Http,
BearerFormat = "JWT",
Scheme = "Bearer"
});
option.AddSecurityRequirement(new OpenApiSecurityRequirement
{
{
new OpenApiSecurityScheme
{
Reference = new OpenApiReference
{
Type=ReferenceType.SecurityScheme,
Id="Bearer"
}
},
new string[]{}
}
});
});
builder.Services.AddAuthentication(opt => {
opt.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
opt.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
})
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = builder.Configuration["JWT:ValidIssuer"],
ValidAudience = builder.Configuration["JWT:ValidAudience"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(builder.Configuration["JWT:Secret"]))
};
});
You configure Swagger generating services when you use AddSwaggerGen in the Program.cs file of your ASP.NET Core application inside the ConfigureServices function..
Step 3: We are going to add AuthController file under the Controllers folder.
public IActionResult Login([FromBody] UserModel user)
{
if (user is null)
{
return BadRequest("Invalid user request!!!");
}
if (userRepository.CheckUserExist(user))
{
var secretKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(configuration["JWT:Secret"]));
var signinCredentials = new SigningCredentials(secretKey, SecurityAlgorithms.HmacSha256);
var tokeOptions = new JwtSecurityToken(
issuer: configuration["JWT:ValidIssuer"],
audience: configuration["JWT:ValidAudience"],
claims: new List(),
expires: DateTime.Now.AddMinutes(60),
signingCredentials: signinCredentials
);
var tokenString = new JwtSecurityTokenHandler().WriteToken(tokeOptions);
return Ok(new AuthResponseModel { Token = tokenString });
}
return Unauthorized();
}
Now, run the project and see how it works.
Download Source Code: GitHub Repository
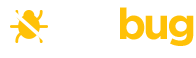